Laravel Caching Techniques: Leveraging Cache for Improved Performance
- mukeshram3
- Jun 23, 2023
- 5 min read
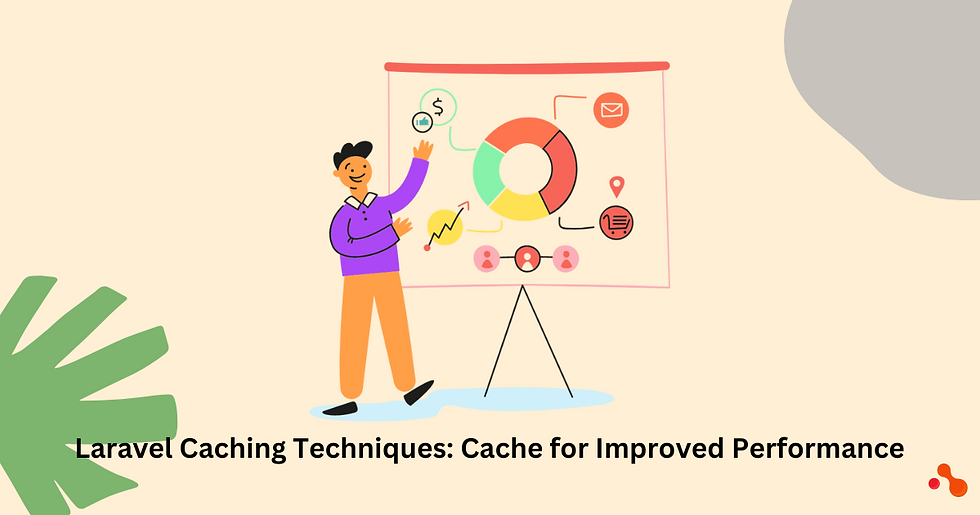
Introduction
Welcome to our blog on Laravel caching techniques! In this article, we'll explore how you can boost the performance of your Laravel development projects by leveraging the power of caching. Whether you're a Laravel developer or looking to hire remote developers for Laravel web development services, understanding caching techniques can greatly improve your application's speed and efficiency. So, let's dive in and discover how you can make the most of Laravel's caching capabilities to optimize your web applications and enhance user experience.
Understanding Caching in Laravel
Caching plays a crucial role in Laravel applications by storing frequently accessed data and speeding up their retrieval process.
There are two types of caching: server-side caching and client-side caching.
Server-side caching involves storing data on the server to avoid time-consuming operations, while client-side caching stores data on the user's device.
Laravel supports various cache drivers, including file, database, Redis, and Memcached, each with advantages and considerations.
File Cache Driver:
This driver stores cached data in files on the server's file system.
Advantages:
Easy to set up and configure.
Suitable for small-scale applications with moderate caching needs.
Considerations:
Slower performance compared to in-memory cache drivers.
Not recommended for large-scale applications with heavy caching requirements.
Database Cache Driver:
With this driver, Laravel stores cached data in a database table.
Advantages:
Allows caching across multiple servers.
Works well for applications already using a database.
Considerations:
Database access can introduce additional latency.
Not as performant as in-memory cache drivers.
Redis Cache Driver:
Redis is an in-memory data structure store, and this driver uses Redis for caching.
Advantages:
High performance due to in-memory storage.
Supports additional features like data expiration and priority queues.
Considerations:
Requires Redis server setup and configuration.
Additional server resource usage compared to file or database drivers.
Memcached Cache Driver:
Memcached is a distributed in-memory caching system, and this driver utilizes Memcached for caching.
Advantages:
Excellent performance with fast data retrieval.
Supports distributed caching across multiple servers.
Considerations:
Requires Memcached server setup and configuration.
Limited features compared to Redis cache driver.
Leveraging Cache in Laravel
Caching is crucial in Laravel development to improve performance and reduce database queries. In this section, we will explore how to leverage cache in Laravel using the cache facade and various techniques for efficient data management. Let's dive in!
Implementing Caching in Laravel:
Laravel provides a powerful cache facade as a gateway to cache operations.
The cache facade allows you to store and retrieve data from the cache effortlessly.
By caching frequently accessed data, you can avoid repetitive database queries and enhance the overall speed of your application.
Cache Operations:
Storing Data: You can store data in the cache using the put method provided by the cache facade. For example:
phpCopy code
cache()->put('key', $data, $minutes);
Retrieving Data: You can use the get method to retrieve data from the cache. If the data is not found, you can provide a default value. For example:
phpCopy code
$data = cache()->get('key', function () {
return 'default value';
});
Cache Tags: Laravel allows you to tag cached items, enabling efficient data management. As a group, you can assign tags to cached items and perform operations on tagged data.
phpCopy code
cache()->tags(['tag1', 'tag2'])->put('key', $data, $minutes);
cache()->tags(['tag1', 'tag2'])->get('key');
Cache Helpers and Global Cache Functions:
Laravel provides cache helpers and global cache functions to simplify cache operations.
Helper Functions:
Cache () - Access the cache facade.
Cache ()->has('key') - Check if an item exists in the cache.
Cache ()->forget('key') - Remove an item from the cache.
Global Cache Functions:
Cache::store('file')->put('key', $data, $minutes) - Specify a different cache store (e.g., file, database, etc.).
Cache::increment('key') - Increment the value of a cached item.
Cache::decrement('key') - Decrement the value of a cached item.
Examples and Code Snippets:
Caching Query Results:
phpCopy code
$users = cache()->remember('users', $minutes, function () {
return DB::table('users')->get();
});
Caching Views:
phpCopy code
return view()->cache($minutes)->make('view.name');
Caching API Responses:
phpCopy code
$response = cache()->remember('api.response', $minutes, function () {
return API::getData();
});
By leveraging cache in Laravel, you can significantly improve the performance of your application by reducing database queries and optimizing data retrieval. Remember to choose appropriate cache strategies based on your application's requirements.
Cache Invalidation and Expiration Strategies
Cache invalidation and expiration are crucial aspects of Laravel development that help improve application performance and user experience. This section will explore the importance of cache invalidation and expiration, techniques for cache invalidation, and various expiration strategies. Here are the key points:
Importance of Cache Invalidation and Expiration:
Caching improves application performance by storing frequently accessed data in memory for quick retrieval.
However, cached data can become outdated, leading to inconsistencies and errors.
Cache invalidation and expiration ensure that stale data is removed from the cache, ensuring data integrity and accuracy.
Techniques for Cache Invalidation:
Manual Clearing: Developers clear the cache when specific events or changes occur, such as data updates or configuration modifications.
Tags-Based Invalidation: Tags are assigned to cached items, allowing developers to invalidate multiple cache entries associated with specific tags.
Cache Keys Management: Developers manage cache keys associated with cached data, allowing precise invalidation of specific items.
Expiration Strategies:
Time-Based Expiration: Cache items are assigned a fixed expiration time and automatically invalidated and refreshed after the specified duration.
Cache Duration Configuration: Developers can configure the cache duration globally or per item, providing control over how long data remains cached.
Cache Revalidation: Instead of immediately invalidating the cache, developers can use revalidation techniques to check if the data has changed before serving it from the cache.
Best Practices and Recommendations:
Identify the critical data that requires caching and implement cache invalidation strategies accordingly.
Use a combination of cache invalidation techniques based on your application's requirements.
Regularly monitor and review the cache expiration settings to ensure optimal performance.
Test cache invalidation and expiration strategies to verify their effectiveness and correctness.
In summary, cache invalidation and expiration are essential for maintaining data consistency and optimizing performance in Laravel development. Developers can effectively manage cache and ensure a smooth user experience using appropriate techniques and strategies.
Advanced Caching Techniques and Optimization
In Laravel development, advanced caching techniques and optimization play a crucial role in improving the performance and efficiency of web applications. Let's explore some key concepts and strategies in a simplified manner:
Advanced Caching Techniques:
Cache Preloading: This technique involves populating the cache with frequently accessed data before users request it. It helps to reduce the response time and enhances user experience.
Cache Warming: In this approach, the cache is prepopulated by simulating user requests or fetching data in the background. It ensures that the cache is already primed with relevant data, reducing the response time for subsequent user requests.
Cache Hierarchies and Strategies:
Edge Caching involves placing caches closer to the users, usually on the edge servers, to minimize latency and improve content delivery speed. Edge caching is often employed in Content Delivery Networks (CDNs) to ensure faster access to static files and assets across geographically distributed locations.
Content Delivery Networks (CDNs): CDNs are networks of geographically distributed servers that store and deliver cached content. Using CDNs, Laravel development services can offload server load, reduce latency, and provide a better user experience.
Optimizations:
Cache Compression: Compressing cached data reduces storage requirements and improves the efficiency of cache usage. It minimizes network bandwidth usage when transmitting cached content.
Cache-Control Headers: By setting appropriate cache control headers, developers can define how long the cached content remains valid and when it needs to be revalidated. This helps control cache behaviour and ensures users receive fresh content when necessary.
Cache Monitoring and Debugging: Implementing monitoring and debugging tools allow developers to track cache utilization, identify performance bottlenecks, and troubleshoot caching issues.
Practical Tips and Examples for Optimizing Cache Usage:
Identify and cache frequently accessed or computationally expensive data.
Use cache tags to group related data and invalidate caches selectively.
Utilize cache expiration and cache invalidation techniques to keep data up to date.
Leverage cache fallback mechanisms to handle cache misses gracefully.
Measure and analyze cache hit rates and response times to identify areas for optimization.
Conclusion
In conclusion, leveraging caching techniques in Laravel development can significantly improve performance. By storing frequently accessed data in cache memory, Laravel reduces the need for time-consuming database queries, resulting in faster response times and better user experience. Caching is a powerful tool that Laravel developers can utilize to optimize web applications. To enhance your Laravel project's performance, consider hiring a Laravel development company or remote developers experienced in Laravel development services. They can help you implement effective caching strategies and boost your application's speed and efficiency.
Comments