Laravel Eloquent Tips and Tricks: Maximizing the Power of Laravel's ORM
- mukeshram3
- Jun 13, 2023
- 6 min read
Introduction
Welcome to our blog on Laravel Eloquent Tips and Tricks! In this article, we will explore ways to maximize the power of Laravel's ORM (Object-Relational Mapping). Whether new to Laravel development or an experienced developer, these tips and tricks will help you enhance your skills and efficiency. You can create robust and scalable web applications by leveraging the potential of Laravel's ORM. So let's dive in and discover how you can make the most out of Laravel's ORM for your development projects.
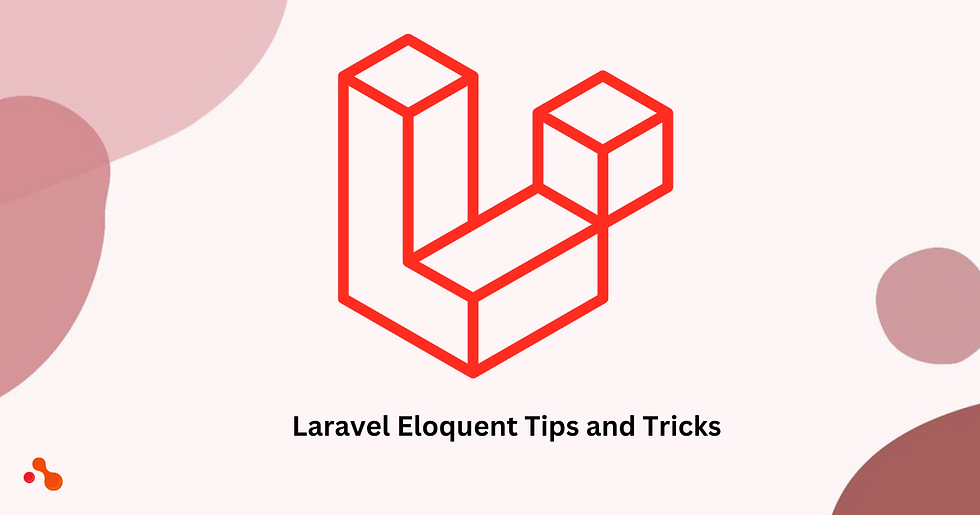
Understanding Eloquent Relationships
Eloquent relationships in Laravel allow you to define the associations between different database tables. These relationships help you establish connections and retrieve related data effortlessly. In this section, we will explore three types of relationships: one-to-one, one-to-many, and many-to-many.
One-to-One Relationship:
This relationship occurs when one record in a table is associated with only one record in another table.
Example: A user has one profile.
Implementation:
In the User model, define the relationship using the hasOne method.
In the Profile model, define the inverse relationship using the belongsTo method.
Challenges:
Ensuring the uniqueness of the relationship.
Retrieving related data efficiently.
One-to-Many Relationship:
This relationship happens when one record in a table is associated with multiple records in another.
Example: A user has many posts.
Implementation:
In the User model, define the relationship using the hasMany method.
In the Post model, define the inverse relationship using the belongsTo method.
Challenges:
Managing cascading deletes or updates.
Optimizing queries when fetching related data.
Many-to-Many Relationship:
This relationship occurs when multiple records in one table are associated with multiple records in another table.
Example: Users have many roles that can be assigned to multiple users.
Implementation:
Create a pivot table that holds the foreign keys of both tables.
In the User model, define the relationship using the belongsToMany method.
In the Role model, define the inverse relationship using the belongsToMany method.
Challenges:
Handling additional attributes in the pivot table.
Working with intermediate models to access related data.
Best Practices:
Choose meaningful names for relationships to enhance code readability.
Utilize eager loading (with the method) to optimize database queries.
Define proper indexes on foreign key columns for better performance.
Leverage the Laravel documentation and community resources for guidance.
Understanding and implementing these relationships in your Laravel development projects allows you to establish efficient associations between your database tables, retrieve related data seamlessly, and maintain a well-structured codebase.
Harnessing Query Scopes for Efficient Queries
In Laravel development, optimizing queries can significantly improve the performance of your web applications. One powerful tool at your disposal is query scopes, which allow you to define reusable query constraints. In this section, we will introduce query scopes, explain how to define and use them, demonstrate their usage with practical examples, and share advanced tips for better performance.
Introducing Query Scopes
Query scopes are a way to encapsulate common query constraints in Laravel.
They provide a convenient and reusable approach to defining specific query conditions.
Using query scopes can make your queries more efficient and reduce code duplication.
Defining and Using Query Scopes
To define a query scope, create a method in your Eloquent model that returns a query builder instance.
Use the where and other query builder methods to define the desired constraints within the method.
For example, you have a User model and want to retrieve all active users. You can define a scope called active like this:
phpCopy code
public function scopeActive($query)
{
return $query->where('status', 'active');
}
To use the query scope, simply chain it onto your queries:
phpCopy code
$activeUsers = User::active()->get();
Demonstrating Usage with Practical Examples
Suppose you have a Laravel development company and want to fetch all projects with a certain budget range. You can define a query scope called budgetRange:
phpCopy code
public function scopeBudgetRange($query, $min, $max)
{
return $query->whereBetween('budget', [$min, $max]);
}
Now you can easily retrieve projects within a specific budget range:
phpCopy code
$projects = Project::budgetRange(1000, 5000)->get();
Advanced Tips for Better Performance
Avoid applying unnecessary constraints in query scopes, as they can impact performance.
Consider eager loading related models within your query scopes using the method to reduce additional queries.
Use index columns appropriately to speed up queries involving frequently used query scopes.
Leverage mechanisms like Laravel's query caching or external caching systems for frequently accessed queries.
By harnessing the power of query scopes, you can optimize your Laravel queries and improve the overall performance of your web applications. Remember to define and use query scopes efficiently, apply them to practical examples, and implement advanced tips to achieve the best results in your Laravel development projects.
Mastering Eager Loading for Efficient Data Retrieval
Eager loading is crucial in reducing queries and improving performance in Laravel development. By fetching related data upfront instead of making separate queries, eager loading significantly reduces the number of database queries needed to retrieve data, resulting in faster and more efficient data retrieval.
Techniques for Eager Loading Relationships:
Define Relationships: First, establish relationships between models using Laravel's eloquent relationships, such as one-to-one, one-to-many, and many-to-many. These relationships define how different models are related to each other.
Utilize Eager Loading Methods: Laravel provides various methods to load relationships eagerly, such as with, withCount, and load. These methods allow you to specify the relationships to be eagerly loaded when querying data.
Example: To eager load the "comments" relationship for a collection of "posts," you can use them with a method as follows:
cssCopy code
$posts = Post::with('comments')->get();
This retrieves all posts along with their associated comments, minimizing additional queries.
Eager Loading Nested Relationships: Laravel also supports eager loading of nested relationships. You can eager load multiple levels of relationships using dot notation. Example: To eager load both the "comments" and "user" relationships for a collection of "posts," you can use the dot notation as follows: cssCopy code $posts = Post::with('comments.user')->get(); This retrieves all posts along with their associated comments and the user who made each comment, optimizing data retrieval.
Tips to Optimize Eager Loading:
Selectively Load Relationships: Avoid eager loading unnecessary relationships to minimize the amount of data fetched. Only load the relationships you actually need to reduce memory usage and improve performance.
Use Lazy Eager Loading: Laravel provides lazy eager loading using the loadMissing method. This method loads the relationships only when they are accessed, allowing you to load relationships on-demand, enhancing efficiency. Example: Instead of eagerly loading all comments for posts, you can use lazy eager loading as follows: phpCopy code $posts = Post::all(); $posts->loadMissing('comments'); This defers loading comments until they are accessed, optimizing resource usage.
Batch Eager Loading: When dealing with large datasets, consider batch eager loading. Split the data into smaller chunks and load relationships in batches to prevent memory overload and improve overall performance.
By mastering eager loading techniques and optimizing its usage, you can enhance the efficiency of data retrieval in Laravel development, leading to faster application performance and better user experience.
Leveraging Eloquent's Advanced Features
Eloquent ORM is a powerful database management tool in Laravel, a popular PHP framework for web development. In this section, we will explore some advanced features of Eloquent and how they can be used to enhance your Laravel development projects. Let's dive in!
Attribute Casting:
Attribute casting allows you to specify the data types of certain model attributes.
This feature helps automatically convert the attribute values from the database to a specific data type.
For example, you can cast a JSON column to an array or a boolean column to a boolean data type.
Accessors:
Accessors allow you to define custom methods on your Eloquent models to retrieve attributes with modified values.
You can define an accessor by prefixing the method name with "get" and suffixing it with the attribute name in StudlyCase.
For instance, if you have a "full_name" attribute, you can define a "getFullNameAttribute" method that returns the full name by combining the first name and last name attributes.
Mutators:
Mutators are similar to accessors but modify the attribute values before storing them in the database.
You can define a mutator by prefixing the method name with "set" and suffixing it with the attribute name in StudlyCase.
For example, if you have a "password" attribute, you can define a "setPasswordAttribute" method that automatically hashes the password before storing it.
Serialization:
Eloquent provides serialization options to convert your model instances into JSON or arrays.
You can use the "toJson" method to convert a model instance to a JSON string or the "toArray" method to retrieve the model's attributes as an array.
This feature is useful when passing data to APIs or storing it in a different format.
Event Observers:
Event observers allow you to listen to specific events on your Eloquent models.
You can define observers to perform actions when creating, updating, or deleting a model occur.
For example, you can define an observer that sends an email notification whenever a new user is created.
These advanced features of Eloquent provide you with flexibility and control over your data handling in Laravel development. Understanding and utilizing these features can greatly enhance the functionality and maintainability of your applications.
Example: Let's consider a scenario where you have a "User" model with a "birth_date" attribute. You can use an accessor to retrieve the user's age based on their birth date and a mutator to ensure the birth date is stored in a specific format.
phpCopy code
class User extends Model
{
// Accessor
public function getAgeAttribute()
{
return Carbon::parse($this->attributes['birth_date'])->age;
}
// Mutator
public function setBirthDateAttribute($value)
{
$this->attributes['birth_date'] = Carbon::parse($value)->format('Y-m-d');
}
}
In this example, accessing $user->age will return the age of the user based on the birth date, and setting $user->birth_date will automatically format and store the birth date in the desired format.
Conclusion
In conclusion, Laravel's Eloquent ORM is a powerful tool for Laravel development. By implementing these tips and tricks, you can maximize the efficiency and effectiveness of your Laravel projects. Whether you're a developer or a business owner looking for Laravel development services, understanding the capabilities of Laravel's ORM can greatly benefit your projects. Consider hiring a reliable Laravel web development company or remote developers to leverage the full potential of Laravel and take your web applications to the next level.
Commentaires