Optimizing Images in Laravel: Reducing File Sizes for Faster Loading
- mukeshram3
- Aug 2, 2023
- 7 min read
Updated: 7 days ago
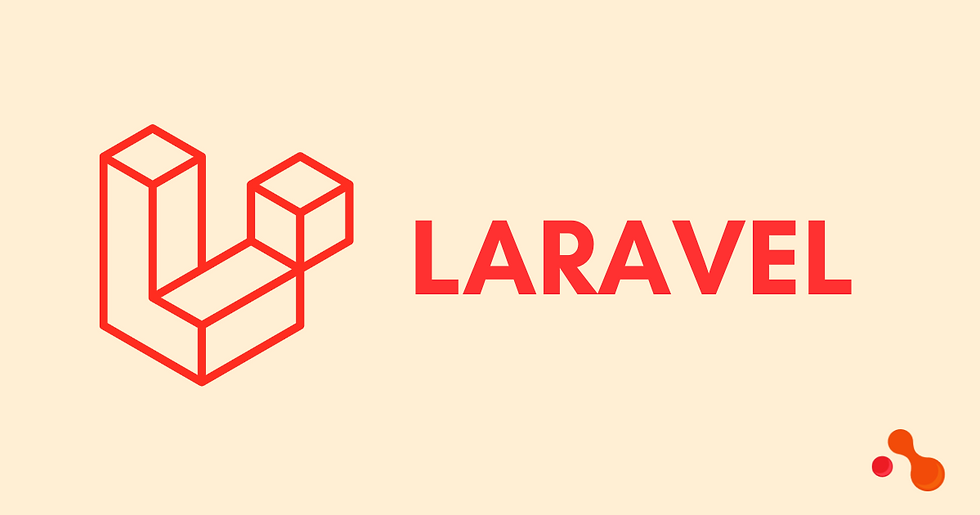
Introduction
In the exciting world of web development, image optimization plays a superhero role. Imagine if your favorite superhero had to carry a heavy backpack everywhere – they wouldn't be as quick and agile, right? Similarly, large image files can slow down your website's speed, making visitors wait longer than they'd like. But fear not! Laravel, a fantastic web development framework, comes to the rescue with tools and techniques that make images lighter and loading faster. In this blog, we'll unravel the magic of reducing image sizes in Laravel, boosting your website's performance.
Understanding Image Optimization
Image optimization is like giving your website a speed boost! It's all about making images on your site load quickly and smoothly. Here's why it matters:
A. What is image optimization?
It's a way to make your images "lighter" without sacrificing quality.
Techniques like resizing, compressing, and choosing the right format help achieve this.
Smaller image sizes mean faster loading times for your website.
B. Why is image optimization crucial for website speed?
Large images slow down your website, frustrating visitors.
Faster loading times make users happy and improve your site's ranking on search engines.
Mobile users, with slower connections, benefit greatly from optimized images.
C. Common issues caused by unoptimized images
Slow page loading: Visitors may leave if your site takes too long to load.
Bad user experience: Blurry or slow-loading images create a poor impression.
High bounce rates: People are more likely to bounce away from a slow website.
In a nutshell, image optimization is like giving your website a makeover – it looks better, loads faster, and keeps visitors coming back for more!
Preparing Your Laravel Project
Before diving into image optimization, make sure your Laravel project is ready to roll. Follow these simple steps to ensure a smooth optimization process:
A. Setting up a new Laravel project (if relevant)
If you're starting from scratch, create a new Laravel project using Laravel development services.
Use the composer create-project command to initiate a fresh Laravel installation.
B. Installing and configuring Intervention Image package
The Intervention Image package is a superhero in Laravel image manipulation.
Install it effortlessly with Composer using composer require intervention/image.
Configure the package in your Laravel app's configuration files.
C. Integrating other image optimization libraries (if applicable)
Depending on your project's needs, consider additional optimization libraries.
Collaborate with a Laravel development company to explore and integrate the best-fit solutions.
Libraries like "Optimus" or "ImageOptimizer" can join the party for extra optimization power.
Remember, these steps set the stage for efficient image optimization. Now you're all set to start shrinking those image file sizes for a blazing-fast website! If you're looking to optimize your project, don't hesitate to hire Laravel developers to guide you through the process.
Techniques for Reducing Image File Sizes
Images play a big role in websites, but large image files can slow things down. Let's dive into techniques to make your images load faster in your Laravel-powered website. Here's how:
A. Choose the Right Format: Pick the best format for your image. JPEG is great for photos, PNG for transparent images, and WebP for modern browsers. This helps keep file sizes in check.
B. Resize Images: Tailor your images to different devices. Big images on small screens waste space and slow load times. Laravel can help resize images to fit perfectly.
C. Compression and Quality: Compression reduces file sizes without sacrificing too much quality. Strike a balance between smaller sizes and good looks.
D. Lazy Loading: Instead of loading all images at once, lazy loading loads images as users scroll. This speeds up the initial page load and saves data for users.
E. Responsive Images: Use srcset and sizes attributes to serve different images based on the user's screen size. This ensures the right image size is loaded, improving both speed and user experience.
Remember, these techniques work wonders for all websites, especially when powered by Laravel. For hassle-free implementation and expert guidance, consider leveraging Laravel development services from a reliable Laravel development company.
Implementation Walk through
A. Step-by-step guide to optimizing images using Intervention Image:
Install the Intervention Image package via Composer in your Laravel project.
Load images using Intervention's methods: Image::make() for opening, and >save() for saving.
Resize images using >resize() and specify dimensions.
Compress images with >encode() and adjust quality settings (higher quality retains more details).
Save the optimized image using >save().
B. Code examples for resizing and compressing images:
phpCopy code
use Intervention\\Image\\Facades\\Image;
// Open, resize, and compress an image
$image = Image::make('path/to/image.jpg')
->resize(800, 600)
->encode('jpg', 80) // 80% quality
->save('path/to/optimized.jpg');
C. Implementing lazy loading and responsive images in Laravel views:
In your view, add the loading="lazy" attribute to <img> tags for lazy loading.
Use the <picture> element to provide different image sources for different screen sizes.
Utilize the srcset and sizes attributes to specify image variants based on device width.
By following these steps, even if you're not a technical expert, you can easily optimize images in your Laravel project using the Intervention Image package. This ensures faster loading times and a better user experience. If you're looking for professional assistance, consider exploring Laravel development services provided by a reputable Laravel development company and hire Laravel developers with expertise in image optimization and web performance.
Automating Image Optimization in Laravel
Automating image optimization in Laravel can significantly improve your website's performance and save time in the development process. Here are some simple steps to achieve this:
A. Utilizing Laravel's Task Scheduling:
Laravel offers a built-in task scheduler that allows you to automate repetitive tasks like image optimization.
Schedule a task to run at specific intervals, optimizing images without manual intervention.
B. Integrating Optimization into the Deployment Pipeline:
Integrate image optimization into your deployment process to ensure that every image is automatically optimized before going live.
This ensures that even new images uploaded by content creators are optimized without extra effort.
C. Monitoring and Benchmarking:
Keep an eye on the performance improvements achieved through image optimization.
Use tools to measure website loading times before and after the implementation to quantify the impact.
By automating image optimization, you enhance the user experience, reduce load times, and create a more efficient development workflow. Consider utilizing Laravel development services or hiring Laravel developers to implement these optimizations and enhance your website's overall performance.
Advanced Techniques for Image Optimization in Laravel
A. WebP Format:
Benefits: WebP is a modern image format that offers high compression and quality, resulting in smaller file sizes. It can significantly improve website loading times.
Browser Support: WebP is supported by most modern browsers, including Chrome, Firefox, and Edge. However, Internet Explorer and some older browsers may not fully support it.
Implementation: To use WebP images in Laravel, you can convert existing images to WebP format using image manipulation libraries or packages like Intervention Image. You should also set up a fallback mechanism for browsers that don't support WebP.
B. CDNs (Content Delivery Networks) for Optimized Image Delivery:
CDNs distribute your website's images across multiple servers worldwide, reducing the distance between users and the server, resulting in faster image delivery.
By integrating a CDN with your Laravel application, images can be served from the nearest CDN edge server to the user, minimizing latency and improving loading speed.
C. Using Caching to Further Enhance Image Loading Speed:
Caching stores previously generated image versions so that they can be quickly retrieved when requested again. This reduces the need for repeated image processing, saving server resources and improving response times.
In Laravel, you can leverage caching mechanisms like Redis or Memcached to cache processed images. This way, subsequent requests for the same image can be served faster, enhancing overall performance.
By implementing these advanced image optimization techniques in your Laravel development services, your website will deliver optimized images, improve loading times, and provide a better user experience. Consider hiring Laravel developers with expertise in image optimization to ensure the successful implementation of these techniques.
Advanced Techniques
A. WebP Format:
WebP is a modern image format developed by Google, known for its smaller file sizes without compromising quality.
Benefits: Faster loading times, reduced bandwidth usage, and improved user experience.
Browser Support: Most modern browsers support WebP, but fallback options are essential for older browsers.
Implementation: Use conversion tools or image libraries (like Intervention Image) to convert and serve images in WebP format for supported browsers.
B. CDNs (Content Delivery Networks):
CDNs are servers distributed worldwide to deliver website content, including images, more efficiently to users.
Benefits: Faster image loading by serving images from servers closer to the user's location.
Implementation: Integrate your Laravel project with a CDN provider (e.g., Cloudflare, Amazon CloudFront) to deliver optimized images.
C. Using Caching:
Caching stores website data temporarily, reducing the need to regenerate content on every request.
Benefits: Faster subsequent page loads by serving cached images.
Implementation: Enable caching in Laravel using caching libraries like "cache-control" headers or Laravel built-in caching mechanisms.
Testing and Measuring Performance
A. Tools for Measuring Performance:
Use tools like Google PageSpeed Insights, Lighthouse, or GTmetrix to assess website performance and identify optimization areas.
B. Conducting Before-and-After Tests:
Measure your website's loading speed before implementing image optimizations.
Apply the image optimization techniques discussed and retest to compare improvements.
C. Interpreting Results and Making Further Optimizations:
Analyze test results and identify areas where further improvements can be made.
Continuously monitor performance and adapt as needed to keep your website loading quickly.
Remember, implementing these advanced techniques will help boost your website's speed, making it more enjoyable for all your users.
Conclusion
In this blog, we've learned why image optimization is crucial for a faster website. Large image files slow down loading times and impact user experience. Fortunately, Laravel offers fantastic tools like Intervention Image package for optimization. Remember to choose the right image format, resize and compress images, and implement lazy loading and responsive images. By automating optimization and testing, your website can achieve remarkable improvements. Embrace these techniques in your Laravel projects to ensure a better user experience and enhanced performance.
Comentários