What are the best practices in Laravel Development?
- mukeshram3
- Apr 17, 2023
- 7 min read
Introduction
Laravel is a very popular framework used to build web-based applications. Many businesses employ it, including Laravel Development Services and Laravel Web Development Company. When you develop using Laravel, the best practices are essential to ensure your code is arranged safely, securely, and effectively. Think of the best practices as adhering to the laws of the road while driving. Like traffic laws will keep you and your passengers secure, implementing the best methods of Laravel development will allow you to stay clear of errors and make the code simpler to maintain.
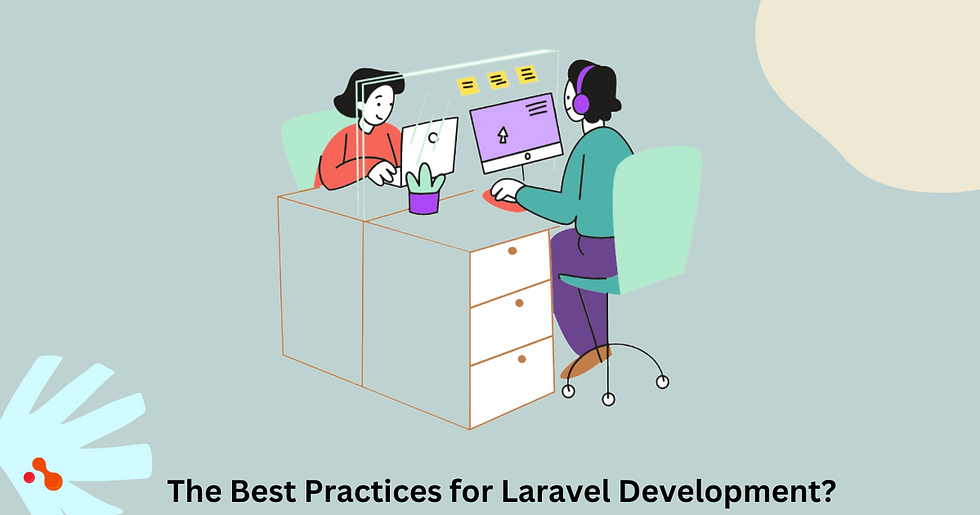
In this blog, we'll discuss the best practices for Laravel development. If you follow these guidelines, you can build high-quality Laravel apps that will be simple to maintain and expand.
Best Techniques for Laravel Development
As a Laravel development, adhering to the best practices is essential to ensure secure and efficient website application creation. Here are some top practices to be aware of:
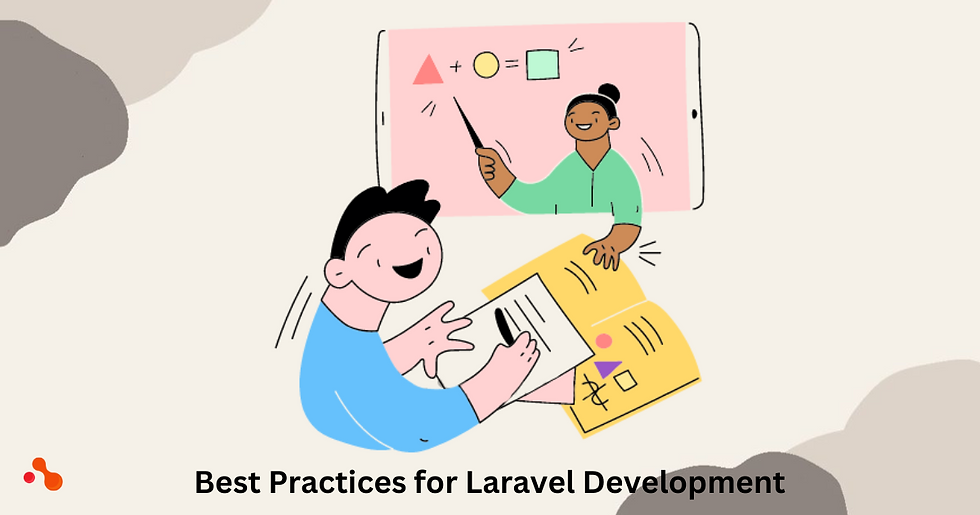
The Code Structure and the Organization
Security
Database Design
Performance Optimization
Testing
Code Structure, Organization, and Code
A well-organized structure for code is essential for Laravel development as it helps the code to understand, read, and maintain. Imagine cleaning your home. Finding things that are located in the correct spot is much simpler!
One method to ensure your Laravel code is organized is using namespaces and a proper directory structure. Namespaces can group related classes, making locating and using them within your code much easier. For instance, if you have an application that manages user authentication, you could put it into the "App/Services/Auth" namespace. It is easy to refer to it in other sections within your application.
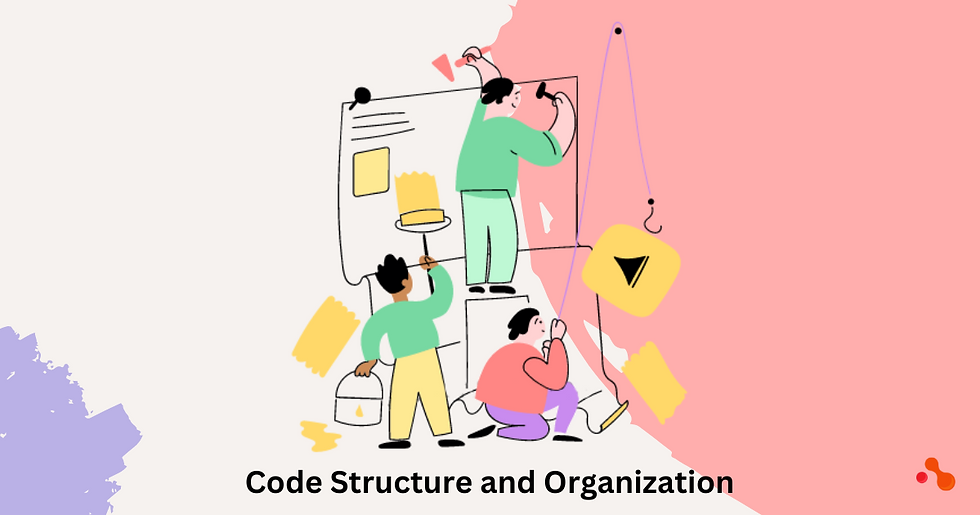
The directory structure of Laravel can also keep things well-organized. As a default feature, Laravel separates your code into folders that include "app," "routes," "views," "controllers," and "models." Each folder has the same code, making locating what you require easier. For instance, all of your models for databases go into the "app/Models" folder, while your view templates go into the "app/Models" directory, while your view templates are all put to the "resources/views" folder.
Here are some helpful tips for organizing your Laravel code:
Use descriptive and clear names for your functions, classes, and variables. This will make it easier for users to comprehend what your code is doing.
Divide the code you write into modules and make use of namespaces to group classes that are related.
Make sure your routes are organized by grouping your routes with similar features. For instance, you could have a set of routes to authenticate users and a separate blog category.
Create subdirectories within your views folders to organize your templates according to the feature or by section. For instance, you could have a "blog" folder with separate directories to store "posts" as well as "categories."
Utilize controllers to tie functionalities that are related. For instance, you may have one called a "UserController," which handles all actions related to users.
With these suggestions, by following these tips, you can build an organized and clean codebase that's easy to use.
Security
Security is the top priority in the development of web applications. As a Laravel development company, it's crucial to be aware of the most common security threats and the best ways to combat these. Here are some tips to consider:
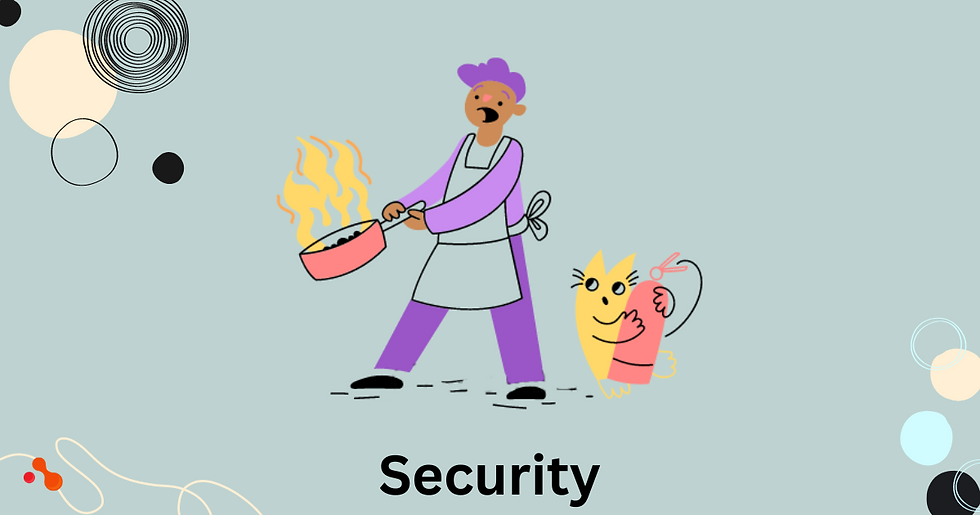
Authorization and Authentication Authentication confirms a person's identity, while authorization determines if a user can access a particular action or resource.
Use Laravel's built-in authentication and authorization tools to ensure the security of your application.
Create strong and unique passwords for your user accounts. Also, encourage users to use two-factor authentication.
Example Use the Laravel class Auth class to manage the authentication of users and user identification.
Encryption: Encryption is the method of encoding data, so authorized individuals can read it. Encryption is a method of protecting sensitive data like passwords and personal data.
Utilize HTTPS to secure data and prevent user information from being stolen.
Example Use Laravel's built-in encryption features to encrypt and decrypt data.
Third-Party PackagesLaravel is a plethora of third-party software that can improve the security of your app.
Utilize packages such as Laravel Passport for secure authentication and API development.
Example Take Laravel's passport installation command to enable OAuth2 authentication for Laravel Passport.
Following these security best practices will help safeguard your data users and help prevent security breaches.
Database Design
The proper design of databases is vital to ensure effective database storage and retrieval during Laravel development. Here's why:
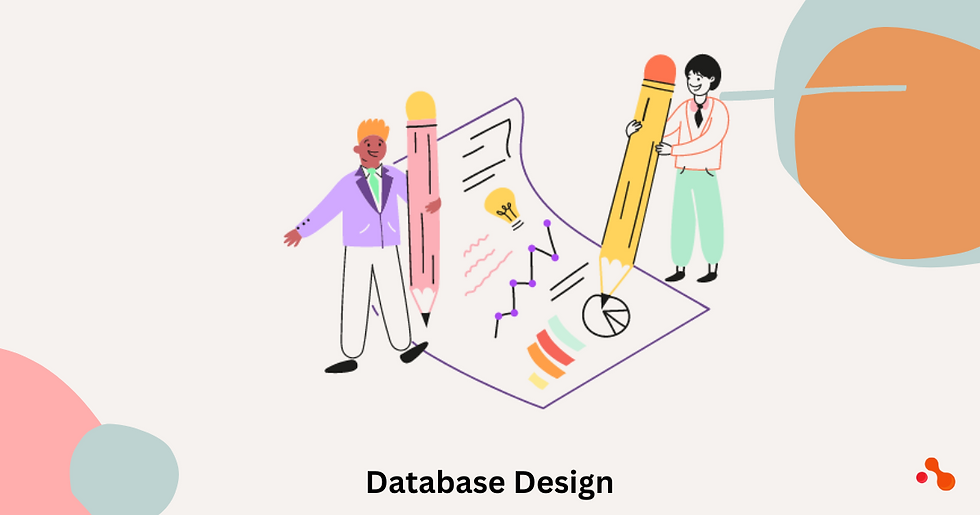
Improved Performance: A proper database design can dramatically improve an application's performance by reducing the amount of redundant data and optimizing the performance of queries.
Data Integrity: A good database design ensures quality and reliability, simplifying, maintaining, and expanding your application.
Simple Maintenance: Well-designed databases are simpler to keep up-to-date and maintain as your application develops.
To ensure a proper design of databases for Laravel development, Here are a few best techniques:
Utilizing Data Migrations in Laravel's built-in migration feature lets you manage your database schema in a version-controlled manner and makes it simple to roll back and update modifications. Utilize the migration feature to control your schema for databases and guarantee uniformity across all environments.
Seeders let you fill your database with the initial data, which makes testing and creating your application much easier. Seeders are used to generate dummy data for testing and to fill databases with the default value.
Use Relationships: Correctly defining relationships between tables will improve the integrity of data as well as simplify database queries. Utilize Laravel's Eloquent ORM built-in to establish the relationships between your models and tables.
Below is an example of how you can create a one-to-many relation of a user model as well as the Post model within Laravel:
KotlinCopy code
class User extends Model
{
Posts for public functions()
{
return $this->hasMany(Post::class);
}
}
class Post extends Model
{
Public functions user()
{
return $this->belongsTo(User::class);
}
}
If you define the relationship between them, users can easily find a user's posts by using $user> or a post's owner by using $post>.
The following best practices will ensure your database's design is efficient, secure, and simple to manage.
The optimization of performance in Laravel Development
Performance optimization is a process that improves the speed and performance of your website. Making improvements to your Laravel website application for speedy loading times, smooth navigation for users, and efficient usage of resources is crucial. Here are some areas to concentrate on to optimize performance:
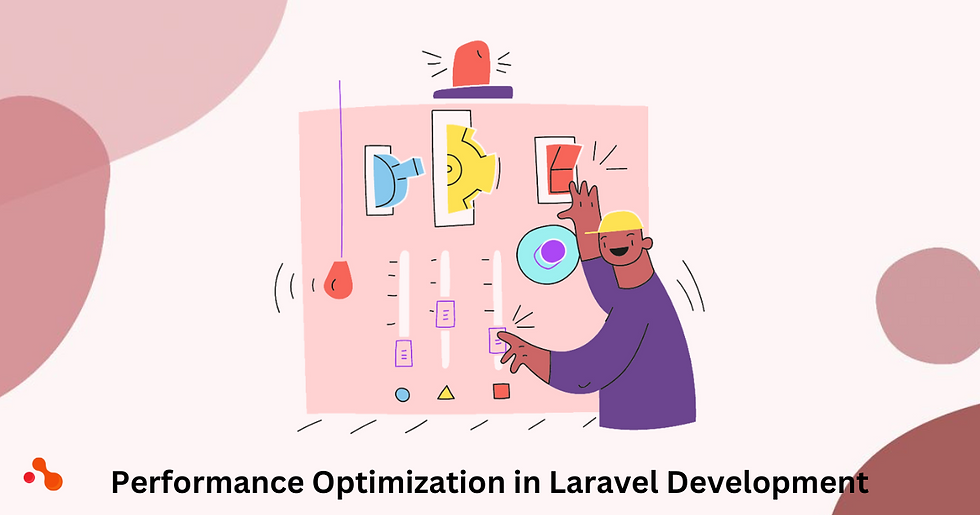
Caching stores frequently-used data in memory, reducing the required database requests. In Laravel, you can use caching to store views, data, and routes. For instance, if you have a page that displays the products available and you want to cache the query that returns the items so that it does not have to be run each time the page loads.
Database Optimization Database optimization can improve the performance of database queries. In Laravel, it is possible to use Eloquent to create efficient queries and use indexes to boost your queries' performance. For instance, if you have a table with many rows, you can create an index for the columns frequently utilized in query queries to increase the execution of queries.
Code optimization Optimization of code is the process that improves the performance of your application code. This could include optimizing loops, reducing the number of database requests, or utilizing caching when appropriate. For instance, if you have a repeatedly running loop, you could make it more efficient using a for loop instead of a for loop.
Laravel provides tools like Laravel Telescope and Blackfire for profiling and debugging to optimize performance. Laravel Telescope is a debugging tool that lets you easily track the application's performance and pinpoint problems with performance. Blackfire is an instrument for profiling that lets you pinpoint the performance bottlenecks in your code.
Performance optimization is essential in Laravel development, concentrating on cache-optimizing databases, optimizing code, and using tools such as Laravel Telescope or Blackfire to ensure your Laravel web application is speedy and effective.
Testing
Testing is a crucial component of Laravel development. It ensures that your code runs as planned and helps identify bugs before they can make it into production. Testing can be classified into three major kinds: unit, feature, or integration testing.
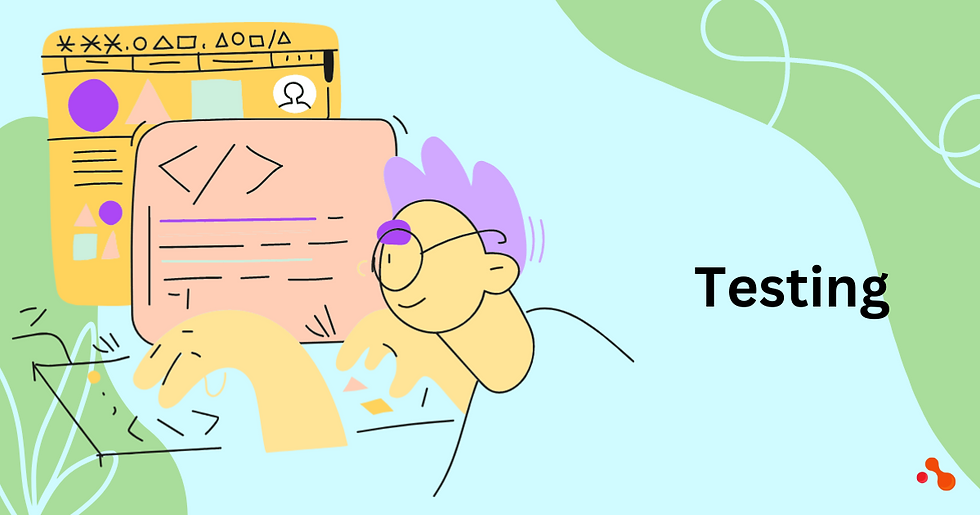
Unit tests: They test individual parts of code, like methods or functions. They verify that each program works according to the plan and doesn't create errors.
Tests for features: They test a feature's completeness or feature within the application. They test that the feature functions exactly as it should and is compatible with other components in the program.
Integration tests test the interaction between various parts of your app. They help verify that all components function in an expected way.
Testing is crucial as it allows you to spot bugs before they cause issues in production. This can save time and money in the long run and guarantees the highest quality Laravel website development.
For testing with Laravel, You can use the built-in testing tools or third-party software such as PHPUnit or Laravel Dusk. PHP Unit is a popular testing framework that runs on PHP and is included in Laravel as a default. Laravel Dusk is an automated test tool that permits users to test their applications within a browser.
For example, let's say you're developing an online store using Laravel. You can create Unit tests for testing specific functions and features that test the full features, such as product search and integration tests to test the interaction between several components, such as the checkout and shopping cart process.
Testing is a vital element of Laravel development. It helps to ensure that the code functions as it should and detects bugs in the early stages. By following the best practices for testing, you will ensure high-quality code and a productive project.
Conclusion
It is essential to follow the best practices in Laravel development firms to ensure that they are efficient and secure, high-quality and safe web application development. You can improve your client or customer outcomes by organizing your code by prioritizing security, maximizing the performance of your application, and thoroughly testing it.
It's crucial to encourage developers to continue studying and enhancing their skills by taking online tutorials, courses, or other sources. Laravel's wealth of documentation is available, and thriving community developers can join to enhance their skills.
Staying current with the best practices and developing and learning as a team is essential. If you do this, you can provide the most efficient Laravel development solutions and assist your clients in reaching their goals.
Other resources to learn and enhance Laravel capabilities include Laracasts and Laracasts, the official Laravel documentation and Laracasts, and the Laravel forum for community members. If you hire Remote developers, getting them to participate in these resources and keep improving their abilities is important.
Commentaires